I love reacting with GIFs, pretty much every chance I get. There’s a ton I still don’t understand about my brain, but I find it very easy to relate a situation to a clip from a show or movie I’ve watched.
This leads me to search the internet for this very specific scene and I often can’t find it, or the quality is bad, maybe the text isn’t correct. This led me to figure out how to generate these myself.
Years ago I had used ffmpeg to do this, and I was fine with continuing this approach since it felt less restrictive. There are a bunch of apps out there that can help you do this easily, but they may have length caps.
I’ve broken this process into two scripts that run on Windows, batch files. This depends on ffmpeg being installed already and accessible from the command line. The first batch file will slice the video into a GIF, I’ve called it make_gif.bat:
@echo off
setlocal
REM Check if FFmpeg is installed
where ffmpeg >nul 2>&1
if %errorlevel% neq 0 (
echo FFmpeg is not installed or not in PATH.
echo Please install FFmpeg and try again.
exit /b
)
REM Check if enough parameters are provided
if "%~4"=="" (
echo Usage: %0 input_video start_time end_time output_gif
exit /b
)
REM Set input parameters
set input_video=%1
set start_time=%2
set end_time=%3
set output_gif=%4
REM Create GIF from video snippet
ffmpeg -i "%input_video%" -ss %start_time% -to %end_time% -vf "fps=10,scale=320:-1:flags=lanczos,split[s0][s1];[s0]palettegen[p];[s1][p]paletteuse" "%output_gif%"
echo GIF creation completed: %output_gif%
Here’s how I use this script:
.\make_gif.bat {video filename} {start time} {end time} {output filename}
An example of the usage:
.\make_gif.bat .\somevideo.mkv 00:08:40.000 00:08:44.150 output.gif
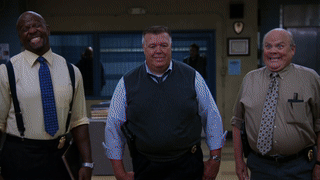
This is good enough for those GIFs that can stand on their own with no text. But I often like to include the text for those who don’t remember the scene as clearly.
Create the following batch file by saving this to a .bat file type in Windows:
@echo off
REM Check if FFmpeg is installed
where ffmpeg >nul 2>&1
if %errorlevel% neq 0 (
echo FFmpeg is not installed or not in PATH.
echo Please install FFmpeg and try again.
exit /b
)
REM Check if enough parameters are provided
if "%~5"=="" (
echo Usage: %0 input_filename start_frame_number end_frame_number text_to_add output_filename [font_size]
exit /b
)
REM Set script parameters
set input_gif=%~1
set start_frame=%~2
set end_frame=%~3
set text=%~4
set output_gif=%~5
set border_width=2
if "%~6"=="" (
set font_size=24
) else (
set font_size=%~6
)
REM Build and run ffmpeg command
ffmpeg -i %input_gif% -vf "drawtext=fontfile=C\\:/Windows/fonts/arial.ttf:text='%text%':fontcolor=white:bordercolor=black:borderw=%border_width%:fontsize=%font_size%:x=(w-text_w)/2:enable='between(n,%start_frame%,%end_frame%)':y=h-th-5,split[s0][s1];[s0]palettegen[p];[s1][p]paletteuse" %output_gif%
REM Check for errors
if errorlevel 1 (
echo Error adding text to gif.
exit /b 1
)
echo Text added to gif successfully.
Here’s how the script is used:
.\add_text_to_gif.bat {input filename} {start frame number} {end frame number} {text added} {output filename} {font size, optional}
An example of the usage:
.\add_text_to_gif.bat output.gif 0 20 "GIF text goes here" output2.gif 20
The frame numbers can be figured out by guessing around the length of the clip as well as the frames per second. I believe the GIFs are generated at 10 frames per second.
It looks silly using the two output filenames but it flows nicely from the command line. I could just change the first output from making the GIF but that doesn’t seem right either.
I hope others find this useful and we get many more GIFs on the internet.
Feel free to leave some suggestions for improvements, or share a GIF you created!
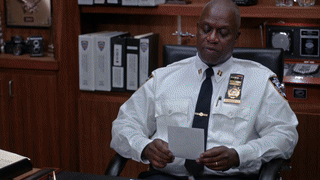
Leave a Reply